React Flow
Published
1. Introduction to React Flow
React Flow is a versatile React library for building node-based applications, from simple diagrams to highly interactive visual editors and data visualizations. It offers customizable node and edge types, built-in components like minimaps and viewport controls, and robust TypeScript support for type safety. By abstracting away much of the complexity of rendering and managing interactive diagrams, React Flow lets developers focus on the unique logic and design of their applications.
Because it’s deeply aligned with React’s component architecture, getting started is straightforward for anyone familiar with React. React Flow also provides plugins for backgrounds, minimaps, controls, panels, node toolbars, and node resizers—making it well-suited for everything from quick prototypes to fully featured production tools.
Key Features of React Flow
- Ease of Use: React Flow handles core interactions—like dragging, zooming, panning, and multiple selections—out of the box.
- Customizability: Developers can create custom node and edge types using standard React components, enabling tailored UIs for any domain.
- Performance: Only nodes and edges that change or appear in the viewport re-render, ensuring efficient handling of large diagrams.
- Built-In Plugins: Pre-packaged components such as a background, minimap, and controls accelerate development and enhance the user experience.
2. Core Concepts and Terminology
React Flow revolves around nodes, edges, and handles. Nodes represent diagram elements, edges depict connections between them, and handles are points on a node that let users draw or receive these connections. The viewport is the area displaying nodes and edges, featuring x, y, and zoom values that let users pan or zoom in and out of the diagram.
- Nodes: Essentially React components that can render any content—text, form elements, data visualizations, etc.
- Edges: SVG paths that link two nodes. React Flow provides default edge types (bezier, smoothstep, step, and straight) and allows custom edge designs.
- Handles: Connection points on a node where edges attach. Placement and styling of handles are fully customizable.
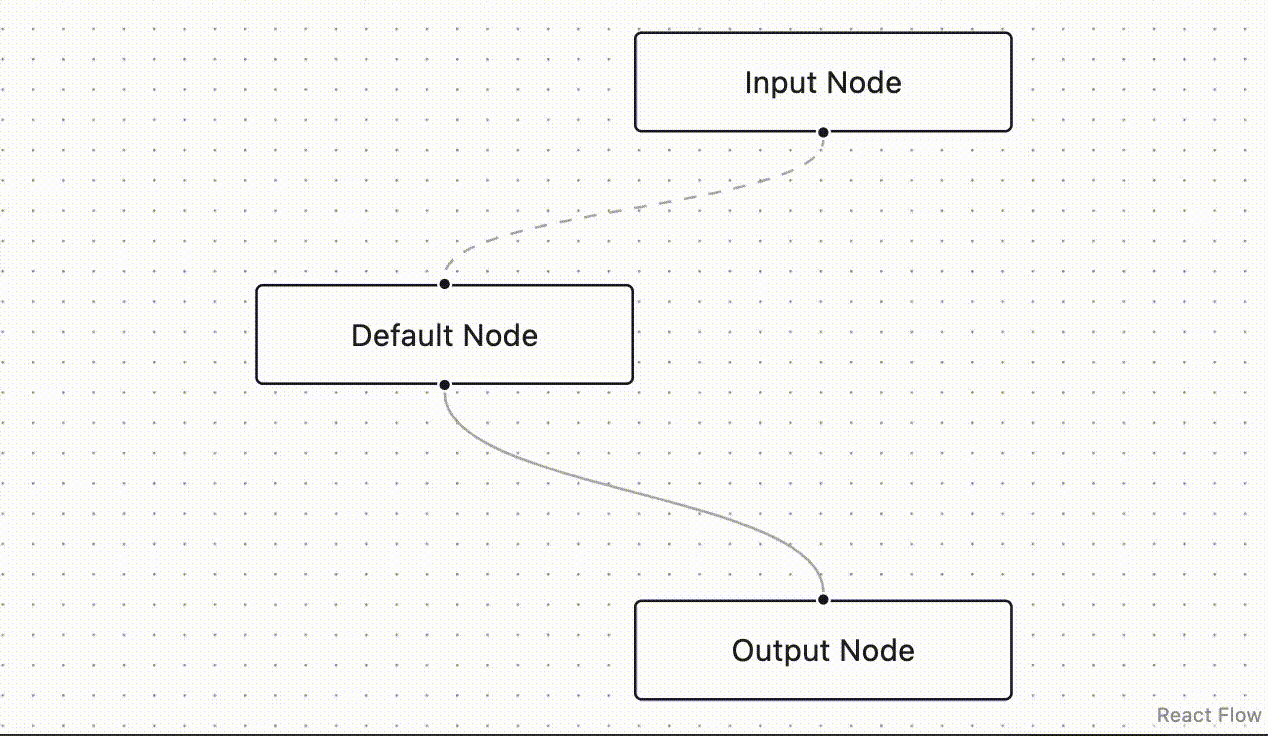
Controlled vs. Uncontrolled Flows
React Flow supports two main state-management approaches:
- Controlled Flow: The application manages nodes and edges state (e.g., via
onNodesChange
andonEdgesChange
). This approach provides fine-grained control, ideal for more complex use cases. - Uncontrolled Flow: React Flow manages state internally. This simpler approach suits basic diagrams where you don’t need to manually track changes.
Controlled flows are typically recommended for most scenarios because they allow you to synchronize the diagram state with other parts of your application.
3. Interactivity and Customization
A key strength of React Flow is its rich interactivity. By default, users can drag nodes, connect edges, and even delete elements. You can easily tap into events—like onNodesChange
, onEdgesChange
, and onConnect
—to implement custom logic or maintain application state.
Because React Flow treats both nodes and edges as React components, you can customize them with CSS or incorporate advanced functionality. Helper functions such as addEdge
streamline tasks like connecting two nodes when the user drags from one handle to another.
Panning and Zooming
Panning (dragging the viewport) and zooming (scrolling) are built-in. You can enable, disable, or modify default behaviors using props like panOnDrag
, zoomOnScroll
, and panOnScrollSpeed
. React Flow also supports specific key-based activation, such as pressing the spacebar to pan—mirroring controls used in many design tools.
Built-in Components
React Flow provides out-of-the-box components to enhance diagrams:
- MiniMap: Displays a small-scale overview of the entire graph, aiding navigation in large diagrams.
- Controls: Adds standard zoom, center, and lock buttons.
- Background: Renders a grid or dots pattern behind the diagram.
- Panel: Positions additional UI elements—like buttons or info panels—on top of the diagram in predefined corners (e.g., top-left, bottom-right).
These components make it easier to build polished, user-friendly node-based interfaces without coding every detail from scratch.
4. Practical Applications and Use Cases
React Flow fits a broad range of node-based UIs:
- Workflow Automation Tools: Visually orchestrate and manage processes by linking nodes representing tasks or data.
- Data Visualization: Represent interconnected data or hierarchical relationships in a more intuitive manner.
- Visual Editors: Provide drag-and-drop tools for building graphs, diagrams, or even music and audio pipelines.
Examples in Action
Teams worldwide use React Flow for everything from small-scale diagramming to complex production workflows. Many open-source and enterprise products rely on its built-in features, while others customize node types and edge connections to craft tailored visual experiences. Its performance, combined with flexible styling and interaction hooks, makes React Flow a strong candidate for large and small projects alike.
Node-Based UIs for React and Svelte
React Flow is part of a broader “xyflow” ecosystem that also includes Svelte Flow—a node-based UI library for Svelte. Both libraries share similar architectural ideas and are maintained by the xyflow team. They are released under the MIT license for open-source use, and a pro version is available for businesses needing advanced features or support.
5. Setting Up a React Flow Project
Setup involves creating a standard React app (via tools like Vite or Create React App) and installing @xyflow/react
. Import the React Flow stylesheet from the library’s dist
folder, then render the ReactFlow
component, supplying it with nodes and edges arrays:
import React from 'react';
import { ReactFlow } from '@xyflow/react';
import '@xyflow/react/dist/style.css';
const initialNodes = [
{ id: '1', position: { x: 0, y: 0 }, data: { label: 'Node 1' } },
{ id: '2', position: { x: 0, y: 100 }, data: { label: 'Node 2' } },
];
const initialEdges = [{ id: 'e1-2', source: '1', target: '2' }];
export default function App() {
return (
<div style={{ width: '100vw', height: '100vh' }}>
<ReactFlow nodes={initialNodes} edges={initialEdges} />
</div>
);
}
Ensure the parent container has explicit width and height, and that you import the necessary CSS for proper rendering.
6. Advanced Techniques and Customization
Beyond basic setups, React Flow supports advanced techniques that let you create unique and specialized UIs.
Custom Nodes and Edges
- Custom Nodes: Implement any React component to display dynamic content (like forms, analytics, or interactive widgets). Use the
nodeTypes
prop to tell React Flow which component to render for each node type. - Custom Edges: Build specialized edge logic with labels, animations, or custom drag handles. Pass these components via the
edgeTypes
prop.
This extensibility caters to highly specific use cases—like specialized diagrams, domain-driven workflows, or dynamic data tools—without losing the library’s core performance benefits.
Plugins and Extensions
React Flow includes several built-in plugins (MiniMap, Controls, Background, Panel), and you can develop your own. They integrate seamlessly with the main ReactFlow
component and can significantly reduce boilerplate for common features:
<ReactFlow nodes={initialNodes} edges={initialEdges}>
<MiniMap />
<Controls />
<Background variant="dots" gap={12} size={1} />
</ReactFlow>
7. Performance Optimization and Best Practices
Although React Flow efficiently re-renders only changed elements, it’s crucial to manage state wisely:
- Minimize Re-renders
Use React’suseMemo
,useCallback
, and stable keys to avoid triggering full re-renders of large graphs. - Keep Node Complexity in Check
Overly complex node components can slow rendering, so consider splitting logic or reducing heavy content. - Avoid Unnecessary State Updates
If your application triggers many changes, isolate or batch updates to prevent performance hits.
Handling Large Datasets
For very large diagrams, consider:
- Conditional Rendering: Only render nodes within the current viewport or those likely to be visible soon.
- Virtualization: Integrate a virtualization library if the diagram must handle tens of thousands of elements.
- Efficient State Management: Avoid frequent full-state updates. Instead, update only the nodes or edges that genuinely change.
Common Pitfalls and Troubleshooting
- Undefined Dimensions: The
ReactFlow
component must be rendered in a container with fixed width and height. - Forgotten CSS Imports: Failing to import
@xyflow/react/dist/style.css
can break node styling. - Over-frequent State Changes: Repeatedly creating new object references or inline functions can hurt performance.
If you encounter specific issues, the xyflow Discord community offers active support, and the official documentation covers many common scenarios.
8. Trends and Community Engagement
React Flow is under active development by the xyflow team, with ongoing improvements influenced by community feedback. The library’s open-source foundation and vibrant user base ensure it remains relevant for complex, modern web applications. Major efforts include performance enhancements, expanded plugin support, and deeper documentation.
The community is highly engaged—sharing tutorials, examples, and helpful libraries. The xyflow team also provides a pro tier, but free licenses for open-source work and student projects foster a collaborative ecosystem where developers can learn from each other and push React Flow’s boundaries.
9. React Flow for Liam
Liam ERD’s implementation showcases how React Flow can handle complex data models. The library’s virtual DOM optimizations and efficient state management made it ideal for large-scale ERDs, ensuring smooth navigation for numerous tables and relationships.
- Edge Animations: While React Flow’s built-in dashed edges added visual appeal, they caused performance bottlenecks with large datasets due to frequent re-renders. The Liam team replaced them with a custom SVG animation to balance fluidity and CPU usage.
- Performance Fine-Tuning: By leveraging motion interpolation and limiting re-renders, they maintained a responsive interface without sacrificing visual clarity.
React Flow’s extensible hooks, active community, and frequent updates make it a strong choice for scalable data-modeling tools, especially when precise rendering control is required. Combining performance, flexibility, and a rich set of built-in features, React Flow simplifies the creation of node-based UIs in React. Whether for simple diagrams or advanced visual editors, its customizable architecture, engaged community, and continuous development ensure it remains a powerful solution for modern, interactive web applications.
References:
Learning Resource: This content is designed to help Liam users learn and grow their skills. For the most current information, please check our official documentation and vendor-specific resources.
GitHub - liam-hq/liam: Automatically generates beautiful and easy-to-read ER diagrams from your database.
Text byTakafumi Endo
CEO of ROUTE06, which develops Liam. After earning his MSc in Information Sciences from Tohoku University, he founded and led an e-commerce startup acquired by a retail company. He also served as an EIR at Delight Ventures.
Last edited on