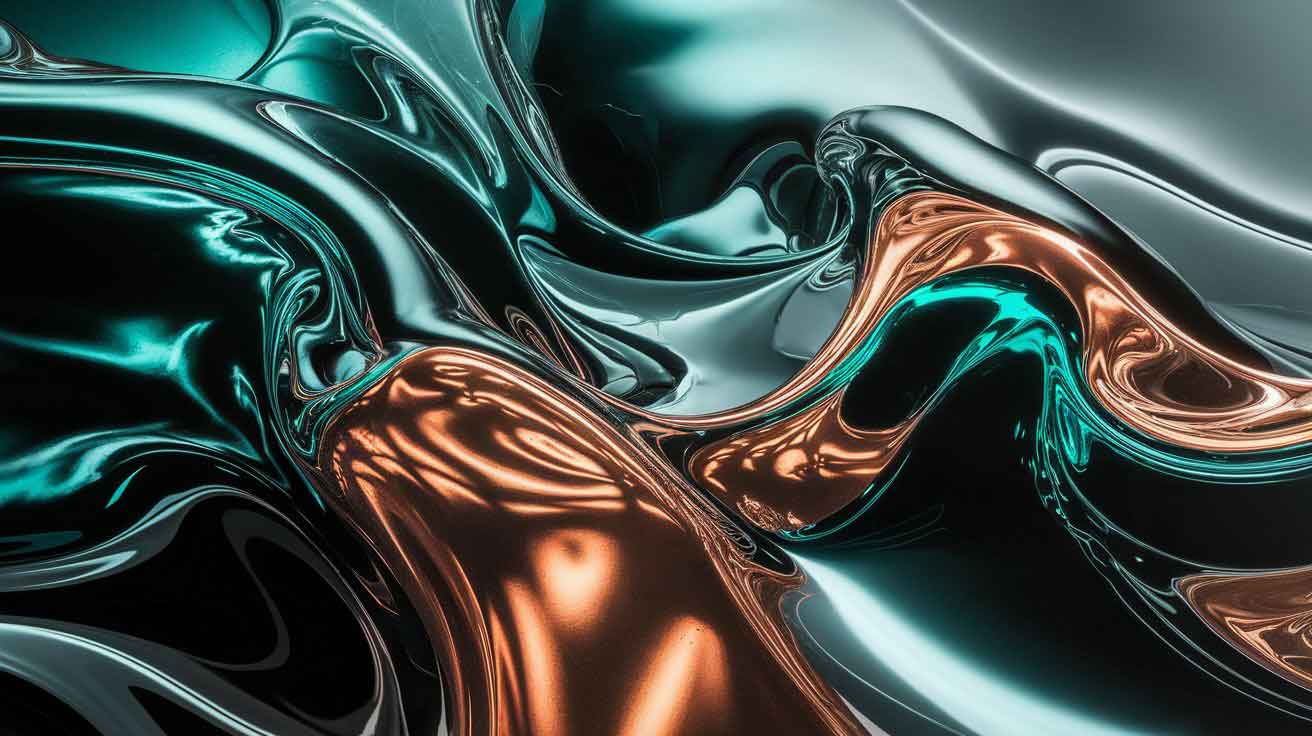
Building REST APIs with PostgreSQL: A Practical Guide to Scalable, and Secure API Development
Text by Takafumi Endo
Published
In today’s tech landscape, building scalable, secure, and high-performance REST APIs is critical for data-driven applications. PostgreSQL has emerged as a powerful choice for backend databases, especially when paired with REST API frameworks like PostgREST, Flask, Encore. This article offers a comprehensive, hands-on guide to creating REST APIs with PostgreSQL, designed for a diverse audience including product managers, engineers, DevOps professionals, and designers.
We start with a foundational understanding of PostgreSQL as an API backend, followed by detailed instructions for setting up REST APIs using different tools and frameworks. Real-world and hypothetical examples illustrate common use cases, such as real-time inventory systems and data synchronization for analytics pipelines. Alongside practical code samples, readers will find insights into performance tuning, security configurations, and operational best practices essential for managing PostgreSQL-based APIs in production.
Advanced topics are also covered, from API rate limiting and caching to asynchronous data handling and JSONB support. By the end, readers will be equipped with the knowledge to implement robust PostgreSQL REST APIs that can scale with application demands, optimize data retrieval, and ensure data integrity and security across client applications.
Introduction
The Evolution of REST APIs with Databases
REST APIs, or Representational State Transfer Application Programming Interfaces, have transformed how applications communicate with databases. Originally conceived as a flexible, lightweight alternative to the more rigid SOAP protocol, REST APIs are now fundamental in enabling seamless data exchange between clients and servers across the web. Unlike traditional approaches where databases were often tightly coupled with application servers, REST APIs decouple these layers, allowing multiple clients—such as web apps, mobile devices, and IoT platforms—to interact with a central database simultaneously and in real time.
This API-centric architecture brought immense benefits, particularly as applications moved to the cloud, where scalability and responsiveness became paramount. REST’s stateless nature ensures that each API request is handled independently, providing a consistent interaction experience. This stateless approach also makes REST an ideal fit for relational databases like PostgreSQL, where structured data can be efficiently queried and managed. PostgreSQL’s capabilities for handling structured data, along with REST’s flexibility, make them a powerful combination, especially in today’s data-rich applications where performance and scalability are non-negotiable.
Why PostgreSQL for REST APIs?
PostgreSQL has grown in popularity not just as an open-source relational database but as a go-to backend for data-intensive applications. It’s an enterprise-class database known for its robustness, scalability, and support for both relational and non-relational (JSON) data types. PostgreSQL’s ACID compliance ensures data integrity across transactions, which is crucial for applications where data consistency is key, such as financial systems or e-commerce platforms.
A unique strength of PostgreSQL is its support for advanced indexing and optimized querying, which are essential for REST APIs handling high query loads and complex data structures. Furthermore, its support for JSON and JSONB data types allows REST APIs to serve semi-structured data efficiently, making it an attractive choice for applications dealing with varied data formats. For instance, PostgREST—a popular tool for generating REST APIs directly from PostgreSQL schemas—takes full advantage of these features, providing a CRUD (Create, Read, Update, Delete) API with minimal configuration. PostgreSQL’s flexibility and performance features make it an ideal foundation for RESTful APIs, allowing developers to meet the demands of multi-client and real-time applications with confidence.
1. Setting Up a PostgreSQL REST API
Choosing the Right Tools for the Job
When setting up a REST API with PostgreSQL as the backend, selecting the right tools is crucial. Each tool brings different strengths depending on the project requirements, team expertise, and deployment scale. Here’s an overview of popular tools for creating PostgreSQL REST APIs, along with key considerations for choosing the best fit.
-
PostgREST: PostgREST is an open-source web server that automatically generates a fully functional REST API based on PostgreSQL schema. It’s ideal for quickly building CRUD (Create, Read, Update, Delete) APIs directly from database tables, providing a zero-code solution that minimizes backend development. With PostgREST, you can spin up an API in minutes, making it a top choice for rapid prototyping and data-driven applications where speed is essential. However, its reliance on database schema means limited flexibility for custom business logic, so it may be best suited for projects where straightforward data operations are sufficient.
-
Flask: Flask, a lightweight Python web framework, is a more customizable option for building REST APIs. Unlike PostgREST, Flask doesn’t auto-generate endpoints, giving developers control over every aspect of the API. Flask is especially valuable when building APIs that require complex business logic, authentication, or custom data processing. Flask pairs well with PostgreSQL for projects where flexibility is crucial, though the trade-off is a more hands-on setup and configuration.
-
Encore: Encore is a backend development framework that simplifies REST API creation, especially for applications deployed in cloud environments. Designed with scalability in mind, Encore offers support for horizontal scaling, making it an ideal choice for high-traffic applications. It also integrates with PostgreSQL and offers built-in DevOps features, such as monitoring and deployment tools, which make it particularly appealing to DevOps teams focused on streamlining infrastructure management.
Key Considerations for Tool Selection
Selecting the right tool depends on several factors:
-
Ease of Setup: For teams needing a fast setup, PostgREST offers minimal configuration and can be deployed quickly. Flask, while flexible, requires more setup time and may not be ideal for teams with limited backend experience.
-
Scalability: High-traffic applications or those anticipating significant growth should consider Encore, which has built-in scalability support. PostgREST is suitable for smaller applications but may need additional configuration for scaling.
-
Flexibility: Flask offers the most flexibility, allowing developers to implement complex business logic and custom endpoints. PostgREST, while efficient, is more restrictive and best for applications with straightforward data interactions.
Each tool has strengths and trade-offs, making it important for teams to align their choice with their specific technical and business requirements.
Building a CRUD API with PostgREST
PostgREST is an efficient choice for teams looking to quickly set up a CRUD API directly on top of PostgreSQL. It provides a RESTful interface for your database by mapping HTTP requests to SQL operations, allowing developers to expose database tables and views as API endpoints with minimal setup. Here’s a straightforward approach to getting started with PostgREST using Docker.
Imagine a B2B company that requires a client portal to manage product information with limited backend resources. By deploying PostgREST, they can quickly make their PostgreSQL data accessible as a REST API without writing backend code. This rapid setup provides immediate access to data for the frontend team, accelerating project timelines and reducing costs associated with custom backend development.
PostgREST is highly suited for applications needing quick database exposure without complex backend logic. However, for more tailored API requirements, consider frameworks like Flask or Encore, which offer greater flexibility in endpoint customization and logic handling.
2. Integrating with Web Frameworks – A Flask API Example
When to Use Flask
Flask, a lightweight Python web framework, is well-suited for building REST APIs that require customization and complex business logic. Unlike tools like PostgREST that automate CRUD endpoints directly from the database, Flask allows developers to define routes, handle intricate data processing, and implement custom features. This flexibility is valuable for projects where predefined database schemas aren’t enough, such as when implementing multi-step authentication, custom error handling, or third-party integrations.
Flask shines when fine-tuned control over each API endpoint is necessary, making it ideal for applications needing more than basic CRUD operations. For instance, an API serving as the backend for a content management system (CMS) or e-commerce site may benefit from Flask's customization options, allowing developers to design detailed filtering, pagination, and complex data relationships that align with user expectations.
Considerations for Flask APIs
While Flask provides flexibility, it also requires more setup and maintenance than automated solutions like PostgREST. Here are some key advantages and limitations to consider:
-
Customization and Flexibility: Flask is ideal when you need full control over each endpoint and API logic. It allows custom code execution, which is necessary for applications requiring more than basic CRUD functionality.
-
Maintenance and Complexity: With Flask, developers must handle routing, error handling, and database interactions manually, which increases setup complexity and ongoing maintenance. This can slow down initial deployment times, making it less ideal for rapid prototyping.
-
Performance and Scalability: Flask apps may require additional configurations, such as load balancers or caching layers, to handle high traffic. For projects with simple CRUD needs and high scalability requirements, PostgREST or Encore might be a more efficient choice.
Flask provides a strong foundation for building custom APIs that require specific functionality and control over data handling. However, for projects needing a faster setup or straightforward database interactions, PostgREST offers a streamlined alternative with reduced code overhead.
4. Security and Operational Considerations
Ensuring Data Security and Access Control
In any API development, security is a primary concern, especially when sensitive data is stored in PostgreSQL databases. Implementing robust security measures for REST APIs includes enforcing access control, securing endpoints, and maintaining logging and monitoring. PostgreSQL offers several built-in features that help secure data access, while additional tools and standards like OAuth and JWT further strengthen API endpoint protection.
Role-Based Access Control (RBAC) in PostgreSQL
PostgreSQL’s Role-Based Access Control (RBAC) allows for fine-grained control over who can access, modify, or query data within the database. By setting up roles and permissions, developers can restrict data access based on user roles, minimizing the risk of unauthorized data exposure.
For instance, in a PostgREST setup, defining a role for anonymous API access (often called web_anon
) and limiting its permissions is essential. Here’s an example of setting up RBAC for an API exposed with PostgREST:
-
Create a Role with Restricted Access:
-- Define a restricted role for anonymous API access CREATE ROLE web_anon NOLOGIN; -- Allow usage of the public schema but restrict sensitive operations GRANT USAGE ON SCHEMA public TO web_anon; GRANT SELECT ON ALL TABLES IN SCHEMA public TO web_anon; -- Revoke potentially sensitive permissions REVOKE INSERT, UPDATE, DELETE ON ALL TABLES IN SCHEMA public FROM web_anon; ALTER ROLE web_anon SET search_path = public;
This setup allows the
web_anon
role to read data but restricts write access, ensuring that sensitive modifications are reserved for authenticated users only. -
Assign Role Permissions via PostgREST Configuration:
By configuring PostgREST to useweb_anon
for anonymous requests, you establish a secure boundary that prevents unauthorized modifications to the database.
RBAC ensures that users only have access to the data they need, a crucial step in protecting API endpoints that interact with sensitive information.
Secure API Endpoints
Beyond RBAC, securing API endpoints is essential for protecting data transmitted over the network. OAuth and JSON Web Tokens (JWT) are two popular methods for managing secure access to REST API endpoints.
-
OAuth: OAuth provides a secure, delegated access method, enabling applications to interact with APIs on behalf of users without exposing user credentials. For example, a frontend app can retrieve an OAuth token to access the API, ensuring that every request is securely authenticated.
-
JWT (JSON Web Tokens): JWTs are compact, URL-safe tokens that verify API requests. After successful authentication, a server issues a JWT, which the client includes in subsequent requests. JWTs ensure that only authorized clients can access specific endpoints, providing a lightweight yet secure access management approach.
With OAuth and JWT, you can set up a layered security strategy that protects against unauthorized access, safeguards user data, and supports scalable authentication for multi-user applications.
Monitoring, Logging, and Maintenance
To keep APIs reliable and responsive, it’s essential to implement monitoring and logging practices. Hevo Data provides a suite of integrated tools for tracking API performance, logging requests, and managing backups, which help teams maintain operational oversight of their REST APIs.
Setting Up Logging and Monitoring with Hevo Data
Logging and monitoring are crucial for API maintenance, helping detect anomalies, prevent downtime, and optimize performance. Hevo Data’s monitoring and logging tools offer centralized insights into API health, performance metrics, and user activity, providing a real-time view of the API’s status.
-
Performance Monitoring: Hevo Data’s built-in performance metrics allow developers and DevOps teams to monitor API response times, error rates, and database load. This helps identify and address performance bottlenecks early, preventing costly downtime.
-
Logging API Requests: Comprehensive logging tracks each API request, making it easier to troubleshoot issues and optimize database queries. By analyzing logs, teams can pinpoint high-traffic endpoints, understand user behavior, and refine API design based on real-world usage.
-
Backup and Recovery: Automated backup features protect against data loss, and Hevo’s backup scheduling provides consistent data snapshots for quick recovery. This reduces the risk of data corruption, ensuring API reliability and continuity.
Error Handling and API Versioning
Effective error handling and API versioning improve user experience and maintain API reliability over time. Here are strategies for each:
-
Error Handling: Clear, consistent error messages enhance usability, especially for frontend developers interacting with the API. Design error responses that are informative and standardized across the API. For example, a 400-level response might indicate input validation issues, while 500-level errors could signal server-side issues. Providing detailed error codes helps developers quickly understand and resolve issues, resulting in a smoother integration experience.
-
API Versioning: As applications evolve, maintaining backward compatibility with older API versions is critical to avoid disrupting users. By implementing API versioning—such as versioning in the URL (
/v1/articles
)—you enable clients to access stable, older versions while developing new features or changes in a different version. This approach minimizes the risk of breaking changes, especially valuable for long-term applications with a diverse user base.
Incorporating comprehensive security and operational practices into PostgreSQL-powered REST APIs not only strengthens data security but also enhances performance, reliability, and scalability. These strategies, from access control to error management, create a robust API ecosystem capable of meeting the needs of modern applications and users alike.
5. Advanced Topics for Further Exploration
To build a high-performance and resilient REST API with PostgreSQL, exploring advanced topics such as asynchronous requests, caching strategies, rate limiting, and JSONB support can significantly enhance the API’s capabilities. These strategies allow developers to tackle real-world challenges like handling high-frequency requests, managing large datasets, and supporting semi-structured data efficiently.
Asynchronous API Requests
Asynchronous requests are essential for APIs that need to handle long-running operations without making users wait. By offloading these operations, such as complex data processing or file uploads, to a background process, developers can keep the API responsive and improve the user experience, especially for frontend applications.
In PostgreSQL-backed applications, developers can implement asynchronous behavior by integrating tools like Celery (commonly paired with Redis as a message broker) or other background worker solutions. Celery is widely compatible with Python-based frameworks like Flask and allows tasks to be queued and processed in the background, improving API responsiveness. These tools enable API requests to initiate tasks that operate independently of the main application thread, allowing clients to receive immediate responses while the backend completes longer-running processes. For instance, in an e-commerce application, asynchronous requests could manage bulk data imports, where the main API responds to the user confirming the task’s initiation, while the import operation proceeds in the background. This setup improves user experience by keeping the interface responsive and delegating intensive operations to background workers.
Custom Caching Strategies
Caching is critical for optimizing performance in REST APIs, particularly for high-traffic endpoints that perform repetitive read operations. PostgreSQL supports multiple caching approaches at different layers, including:
-
Database-Level Caching: PostgreSQL's caching mechanisms, like
pg_prewarm
for warming up frequently accessed data in memory, ensure that commonly queried data is readily available. This reduces disk I/O and speeds up responses for data-heavy endpoints. -
Application-Level Caching: Tools such as Redis or Memcached can cache query results in memory, reducing the need to repeatedly hit the database. For instance, caching the results of a popular product list in Redis can minimize database load while delivering faster response times to users.
-
Client-Side Caching: Developers can leverage HTTP headers (e.g.,
Cache-Control
andETag
) to control how responses are cached on the client side. For APIs serving public, rarely changing data, setting a longer cache duration reduces server load and latency for users.
By implementing these caching layers, developers can achieve a high-performance API capable of handling frequent requests without overloading the PostgreSQL database.
API Rate Limiting and Throttling
Rate limiting is an essential feature for any public-facing API. It protects the backend from abuse and manages load effectively by restricting the number of requests a client can make in a given timeframe. PostgreSQL-backed APIs can implement rate limiting through:
-
API Gateways: Solutions like Kong or AWS API Gateway provide built-in rate limiting and throttling controls, which restrict client requests based on predefined rules. These platforms allow API providers to set rate limits per user or IP address, helping to prevent abuse and manage costs.
-
Custom Middleware: For a more customizable approach, developers can implement rate-limiting middleware directly within their API code (e.g., using Flask with
Flask-Limiter
). This middleware tracks request counts in a caching layer like Redis, allowing the API to reject requests that exceed the allowed threshold.
For instance, a public API might limit users to 100 requests per hour to prevent a single client from overwhelming the system. Rate limiting is particularly useful for APIs that support multiple third-party integrations or client applications, where usage spikes can create sudden load increases.
Exploring JSONB Support in PostgreSQL
PostgreSQL’s JSONB
data type is a powerful feature for APIs handling semi-structured or unstructured data. Unlike traditional relational columns, JSONB
fields allow developers to store JSON documents directly in the database while benefiting from indexing, which enables fast querying and filtering.
The JSONB
data type is ideal for APIs where data structures may vary across records. For example, a social media application might store user profiles with fields that differ for each user (e.g., hobbies, interests, and contact methods). By storing these profiles in a JSONB
column, the API can retrieve data efficiently without needing a rigid schema, while PostgreSQL’s indexing ensures that even complex JSON queries perform well.
Furthermore, JSONB
is well-suited for APIs that need to support evolving data schemas, as the flexible structure accommodates new fields without requiring database migrations. This makes it a valuable tool for modern applications, especially those that manage extensive metadata or allow for user-generated content.
Conclusion
Summary of Key Takeaways
Creating a high-performance, scalable, and secure REST API with PostgreSQL requires a balance of the right tools, best practices, and careful consideration of each layer of the API architecture. Key steps include:
-
Tool Selection: Understanding the strengths of various tools like PostgREST, Flask, Encore, and Hevo Data can help tailor the API stack to the project’s needs. While PostgREST excels at quickly exposing database tables, Flask provides flexibility for custom business logic, and Encore offers scalability features well-suited for high-traffic applications.
-
Database Setup and Configuration: Setting up PostgreSQL with role-based access control (RBAC) ensures security from the outset, while careful data modeling, indexing, and schema design contribute to efficient data querying and retrieval.
-
Advanced API Features: Implementing advanced capabilities like asynchronous processing, custom caching, rate limiting, and JSONB support can elevate API performance and meet the demands of real-world applications.
-
Security and Monitoring: API security is essential for protecting data. Using OAuth, JWT, logging, and monitoring tools like Hevo Data ensures secure and reliable operations over time, while well-defined error handling and versioning improve API resilience.
Each of these steps ensures the API not only meets functional requirements but also performs efficiently, scales with user demands, and maintains data security.
Choosing the Right Approach
When deciding on the right approach for your PostgreSQL-based API, it’s essential to assess:
-
Project Requirements: For simple, CRUD-focused applications, PostgREST provides a quick solution with minimal code. For projects with complex data handling and custom logic needs, Flask or Encore may be better suited due to their flexibility.
-
Scalability Needs: Consider tools with built-in scalability features like Encore if you expect high traffic or need horizontal scaling. For applications with moderate traffic but frequent read operations, caching strategies and PostgreSQL’s indexing can provide performance boosts.
-
Team Expertise: Teams with deep SQL and backend experience may benefit from the control Flask provides, whereas those looking for a low-code or rapid deployment solution might find PostgREST or Hevo Data more efficient.
The choice of tools and configurations ultimately depends on the project’s scale, required flexibility, and available technical expertise.
References:
- Astera | What’s a PostgreSQL REST API and How to Create One
- MakeUseOf | How to Build a REST API with Flask and Postgres
- Hevo Data | PostgreSQL REST API Connection: 4 Easy Methods
- Marmelab | Create a CRUD API in Minutes with PostgREST
- Encore | Building a REST API with Encore
- Flask | Using Celery with Flask
Please Note: This article reflects information available at the time of writing. Some code examples and implementation methods may have been created with the support of AI assistants. All implementations should be appropriately customized to match your specific environment and requirements. We recommend regularly consulting official resources and community forums for the latest information and best practices.
Text byTakafumi Endo
Takafumi Endo, CEO of ROUTE06. After earning his MSc from Tohoku University, he founded and led an e-commerce startup acquired by a major retail company. He also served as an EIR at a venture capital firm.
Last edited on
Categories
- Knowledge
Tags
- API
- RESTful
- Database